Множество:
Массив - это набор элементов, хранящихся в непрерывных ячейках памяти. Идея состоит в том, чтобы хранить вместе несколько предметов одного типа. Это упрощает вычисление положения каждого элемента, просто добавляя смещение к базовому значению, то есть к месту в памяти первого элемента массива (обычно обозначаемого именем массива).
Схематическое изображение массива приведено ниже:
Программа 1:
Ниже приведена иллюстрация одномерного массива:
C ++ 14
#include <bits/stdc++.h> using namespace std; int main() {
int arr[] = { 6, 10, 5, 0 };
for ( int i = 0; i < 4; i++) {
cout << arr[i] << " " ;
} return 0;
} |
Джава
class GFG{ public static void main(String[] args) {
int arr[] = { 6 , 10 , 5 , 0 };
for ( int i = 0 ; i < 4 ; i++)
{
System.out.print(arr[i] + " " );
}
} } |
Python3
if __name__ = = '__main__' :
arr = [ 6 , 10 , 5 , 0 ];
for i in range ( 0 , 4 ):
print (arr[i], end = " " );
|
C #
using System; class GFG{
public static void Main(String[] args)
{
int [] arr = {6, 10, 5, 0};
for ( int i = 0; i < 4; i++)
{
Console.Write(arr[i] + " " );
}
}
} |
Программа 2:
Ниже приведена иллюстрация 2D-массива:
Карта:
Карта - это ассоциативный контейнер, в котором элементы хранятся в отображенном виде. Каждый элемент имеет значение ключа и сопоставленное значение. Никакие два сопоставленных значения не могут иметь одинаковые ключевые значения.
Схематическое изображение карты приведено ниже:
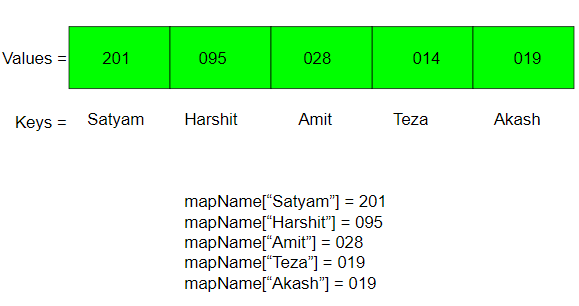
Программа 1:
Ниже приведена иллюстрация карты:
C ++
#include <bits/stdc++.h> using namespace std; int main() {
map< int , int > gquiz1;
gquiz1.insert(pair< int , int >(1, 40));
gquiz1.insert(pair< int , int >(2, 30));
gquiz1.insert(pair< int , int >(3, 60));
map< int , int >::iterator itr;
cout << "
The map gquiz1 is :
" ;
cout << " KEY ELEMENT
" ;
for (itr = gquiz1.begin();
itr != gquiz1.end(); ++itr) {
cout << ' ' << itr->first
<< ' ' << itr->second
<< '
' ;
}
return 0;
} |
Джава
import java.util.*; class GFG{ public static void main(String[] args) {
HashMap<Integer,
Integer> gquiz1 = new HashMap<Integer,
Integer>();
gquiz1.put( 1 , 40 );
gquiz1.put( 2 , 30 );
gquiz1.put( 3 , 60 );
Iterator<Map.Entry<Integer,
Integer>> itr = gquiz1.entrySet().
iterator(); System.out.print( "
The map gquiz1 is :
" );
System.out.print( "KEY ELEMENT
" );
while (itr.hasNext())
{
Map.Entry<Integer,
Integer> entry = itr.next();
System.out.print( ' ' + entry.getKey()
+ " " + entry.getValue()+ "
" );
}
} } |
Python3
if __name__ = = '__main__' :
gquiz1 = dict ()
gquiz1[ 1 ] = 40
gquiz1[ 2 ] = 30
gquiz1[ 3 ] = 60
print ( "
The map gquiz1 is : " )
print ( "KEY ELEMENT" )
for x, y in gquiz1.items():
print (x, " " , y)
|
C #
using System; using System.Collections.Generic; class GFG{ public static void Main(String[] args) {
Dictionary< int ,
int > gquiz1 = new Dictionary< int ,
int >();
gquiz1.Add(1, 40);
gquiz1.Add(2, 30);
gquiz1.Add(3, 60);
Console.Write( "
The map gquiz1 is :
" );
Console.Write( " KEY ELEMENT
" );
foreach (KeyValuePair< int ,
int > entry in gquiz1)
{
Console.Write( " " + entry.Key +
" " + entry.Value + "
" );
}
} } |
Выход: Карта gquiz1:
КЛЮЧЕВОЙ ЭЛЕМЕНТ
1 40
2 30
3 60
Программа 2:
Ниже представлена неупорядоченная карта:
C ++ 14
#include <bits/stdc++.h> using namespace std; int main() {
unordered_map<string, int > umap;
umap[ "GeeksforGeeks" ] = 10;
umap[ "Practice" ] = 20;
umap[ "Contribute" ] = 30;
for ( auto x : umap)
cout << x.first << " "
<< x.second << endl;
return 0;
} |
Джава
import java.util.*; class GFG{ public static void main(String[] args) {
HashMap<String,
Integer> umap = new HashMap<>();
umap.put( "GeeksforGeeks" , 10 );
umap.put( "Practice" , 20 );
umap.put( "Contribute" , 30 );
for (Map.Entry<String,
Integer> x : umap.entrySet())
System.out.print(x.getKey() + " " +
x.getValue() + "
" );
} } |
C #
using System; using System.Collections.Generic; class GFG{
public static void Main(String[] args)
{
Dictionary<String, int > umap = new Dictionary<String,
int >();
umap.Add( "Contribute" , 30);
umap.Add( "GeeksforGeeks" , 10);
umap.Add( "Practice" , 20);
foreach (KeyValuePair<String, int > x in umap)
Console.Write(x.Key + " " + x.Value + "
" );
}
} |
Выход:Contribute 30
GeeksforGeeks 10
Practice 20