Программа на Java для вставки нового узла в середину кругового связного списка
Опубликовано: 21 Января, 2022
Учитывая круговой связанный список, задача состоит в том, чтобы добавить новый узел в середину списка. Рассмотрим следующий круговой связанный список:
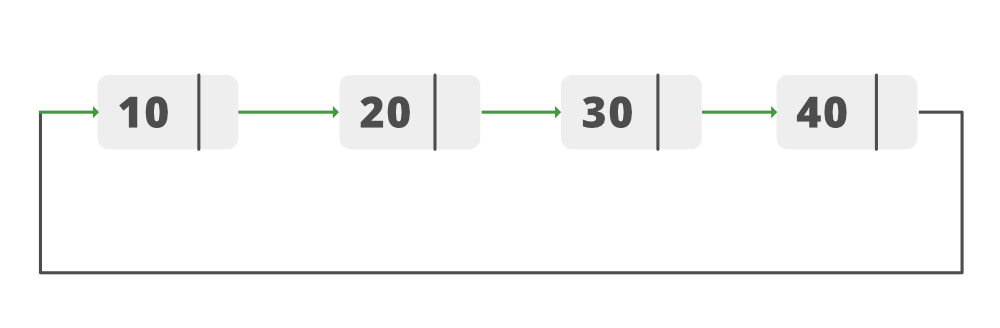
Список перед вставкой
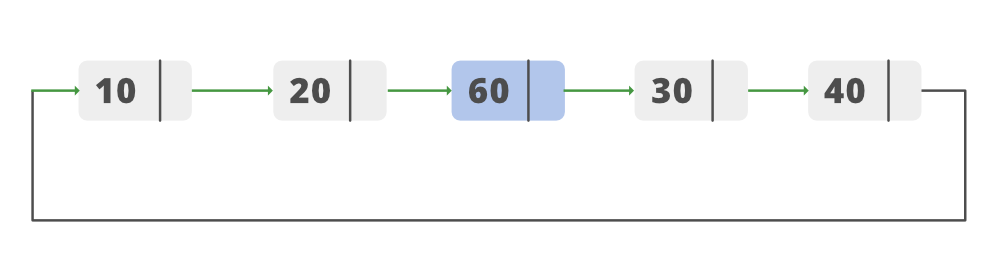
Список после вставки
- Создайте новый узел (New_node).
- Проверьте пустой список. Если список пуст, вставьте узел как заголовок.
- Для непустого списка рассчитайте длину списка.
- Создайте переменную mid и сохраните в ней среднюю длину.
- Создайте два узла: «Временный» и «Текущий».
- Теперь перемещайтесь по списку, пока Temporary не достигнет середины списка, используя переменную mid.
- Вставьте New_node после Current.
- Сделайте Current.next указателем на новый узел и New_node.next на временный.
- Узел вставлен.
Below is the implementation of the above approach:
Java
// Java Program to Insert a New Node at // the Middle of the Circular Linked List import java.io.*; public class GFG { // Stores Information about Node of List public class Node { int data; Node next; public Node( int data) { this .data = data; } } // Declaring Head of the Node public Node head_of_node = null ; // A last pointer to help append values to our list public Node last = null ; // keep count of size of the list public int length_of_list; // Add method adds values to the end of the list public void add( int data) { Node newNode = new Node(data); if (head_of_node == null ) { head_of_node = newNode; last = newNode; newNode.next = head_of_node; } else { last.next = newNode; last = newNode; last.next = head_of_node; } // keep count of size of list length_of_list++; } // Method to insert Node in the middle of the list public void Insert_In_Middle( int data) { // creating a new node that is to be inserted Node New_node = new Node(data); // check for empty list if (head_of_node == null ) { head_of_node = New_node; last = New_node; New_node.next = head_of_node; } // If the list is not empty else { // Declaring nodes Node temporary; Node current; // Checking if the length of list // if even or odd int mid_point_check = (length_of_list % 2 ); // mid point for even length if (mid_point_check == 0 ) { length_of_list = length_of_list / 2 ; } // mid point for odd length else { length_of_list = (length_of_list + 1 ) / 2 ; } // temporary points to the head of list temporary = head_of_node; current = null ; // loop till we reach the mid point while (length_of_list > 0 ) { // make current point to the previous node current = temporary; temporary = temporary.next; length_of_list -= 1 ; } // make the previous node point to the new node current.next = New_node; // make the new_node.next point to temporary New_node.next = temporary; } // increasing the length of list after insertion of // new node length_of_list++; } // Print_list method iterates through the list and // prints the values stored in the list public void Print_List() { Node current = head_of_node; if (head_of_node == null ) { System.out.println( "Your list is empty" ); } else { do { System.out.print( " " + current.data); current = current.next; } while (current != head_of_node); System.out.println(); } } // Driver code public static void main(String[] args) { GFG circular_list = new GFG(); circular_list.add( 10 ); circular_list.add( 20 ); circular_list.add( 30 ); circular_list.add( 40 ); System.out.print( "Original List --> " ); circular_list.Print_List(); circular_list.Insert_In_Middle( 60 ); System.out.print( "List after Inserting --> " ); circular_list.Print_List(); } } |
Output
Original List --> 10 20 30 40 List after Inserting --> 10 20 60 30 40
Вниманию читателя! Не прекращайте учиться сейчас. Освойте все важные концепции DSA с помощью самостоятельного курса DSA по приемлемой для студентов цене и будьте готовы к работе в отрасли. Чтобы завершить подготовку от изучения языка к DS Algo и многому другому, см. Полный курс подготовки к собеседованию .
Если вы хотите посещать живые занятия с отраслевыми экспертами, пожалуйста, обращайтесь к Geeks Classes Live и Geeks Classes Live USA.