Учитывая массив из N элементов, задача состоит в том, чтобы найти Сумму из N элементов без использования циклов (for, while & doWhile) и рекурсии.
Примеры:
Ввод : arr [] = {1, 2, 3, 4, 5}
Выход : 15
Ввод : arr [] = {10, 20, 30}
Выход : 60
Рекомендуется: сначала попробуйте свой подход в {IDE}, прежде чем переходить к решению.
Подход: для решения этой проблемы можно использовать операторы безусловного перехода.
Заявления о безусловном прыжке:
Операторы перехода прерывают последовательное выполнение операторов, поэтому выполнение продолжается в другой точке программы. Переход уничтожает автоматические переменные, если пункт назначения перехода находится за пределами их области видимости. Есть четыре оператора, которые вызывают безусловные переходы в C: break, continue, goto и return.
Для решения этой конкретной проблемы может быть полезен оператор goto.
Заявление goto :
Оператор goto - это оператор перехода, который иногда также называют оператором безусловного перехода. Оператор goto может использоваться для перехода из любого места в любое место внутри функции.
Синтаксис :
Syntax1 | Синтаксис2
----------------------------
метка перехода; | метка:
. | .
. | .
. | .
этикетка: | метка перехода;
В приведенном выше синтаксисе первая строка сообщает компилятору перейти к оператору, помеченному как метка, или перейти к нему. Здесь метка - это определяемый пользователем идентификатор, который указывает целевой оператор. Оператор, следующий сразу за меткой: - это оператор назначения. Метка «метка:» также может стоять перед меткой перехода; в приведенном выше синтаксисе.
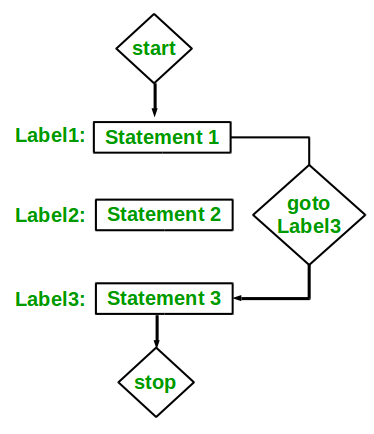
Ниже представлена реализация описанного выше подхода:
C ++
#include <iostream> using namespace std; int operate( int array[], int N) { int sum = 0, index = 0;
label: sum += array[index++]; if (index < N) {
label; goto
}
sum; return
} int main() {
int N = 5, sum = 0;
int array[] = { 1, 2, 3, 4, 5 };
sum = operate(array, N);
cout << sum;
} |
C
#include <stdio.h> int operate( int array[], int N) { int sum = 0, index = 0;
label: sum += array[index++]; if (index < N) {
label; goto
}
sum; return
} int main() {
int N = 5, sum = 0;
int array[] = { 1, 2, 3, 4, 5 };
sum = operate(array, N);
printf ( "%d" , sum);
} |
Джава
class GFG {
static int operate( int array[], int N)
{
int sum = 0 , index = 0 ;
while ( true )
{
sum += array[index++];
if (index < N)
{
continue ;
}
else
{
break ;
}
}
sum; return
}
public static void main(String[] args)
{
int N = 5 , sum = 0 ;
int array[] = { 1 , 2 , 3 , 4 , 5 };
sum = operate(array, N);
System.out.print(sum);
}
} |
Python3
def operate(array, N) : Sum , index = 0 , 0
while ( True ) :
Sum + = array[index]
index + = 1
if index < N :
continue
else :
break
return Sum
N, Sum = 5 , 0 array = [ 1 , 2 , 3 , 4 , 5 ] Sum = operate(array, N) print ( Sum ) |
C #
using System; class GFG { static int operate( int [] array, int N) { int sum = 0, index = 0;
label: sum += array[index++]; if (index < N)
{
label; goto
}
sum; return
} public static void Main() {
int N = 5, sum = 0;
int [] array = { 1, 2, 3, 4, 5 };
sum = operate(array, N);
Console.Write(sum);
} } |
PHP
<?php function operate( $array , $N ) { $sum = 0;
$index = 0;
label:
$sum += $array [ $index ++];
if ( $index < $N )
{
label; goto
}
return $sum ;
} $N = 5; $array = array (1, 2, 3, 4, 5); echo operate( $array , $N ); ?> |
Javascript
<script>
function operate(array, N)
{
let sum = 0, index = 0;
while ( true )
{
sum += array[index++];
if (index < N)
{
continue ;
}
else
{
break ;
}
}
sum; return
}
let N = 5, sum = 0;
let array = [ 1, 2, 3, 4, 5 ];
sum = operate(array, N);
document.write(sum);
</script> |
Хотите узнать о лучших видео и практических задачах, ознакомьтесь с базовым курсом C ++ для базового и продвинутого уровня C ++ и курсом C ++ STL для базового уровня плюс STL. Чтобы завершить подготовку от изучения языка к DS Algo и многому другому, см. Полный курс подготовки к собеседованию .